by Contributed | Sep 24, 2020 | Azure, Technology, Uncategorized
This article is contributed. See the original author and article here.
TodoMVC is a very well known (like ~27K GitHub stars known) application among developers as it is a really great way to start to learn a new Model-View-Something framework. It has plenty of samples done with different frameworks, all implementing exactly the same solution. This way is very easy to compare them against each other and see what is the one you prefer. Creating a To-Do App is easy enough, but not too easy, to be the perfect playground to learn a new technology.

The only issue with TodoMVC project is that it “only” focus on front-end solutions. What about having a full-stack implementation of the TodoMVC project with also back-end API and a database? Well it turns out that there is also an answer for that: Todo-Backend. There are more than 100 implementations available! Pretty cool, uh?
If you want to have a test run building a full-stack solution using a new technology stack you want to try, you are pretty much covered.
Full Stack with Azure Static Web Apps, Node, Vue and Azure SQL
Lately I was intrigued by the new Azure Static Web Apps that promises an super-easy Azure deploy experience, integration with Azure Function and GitHub Actions, and ability to deploy and manage a full-stack application in just one place, so I really wanted to try to take the chance to create a 100% serverless TodoMVC full stack implementation using:
-
Vue.Js for the frontend as I find it really really cool and powerful;
-
Azure Static Web Apps as I can manage the full-stack app just from one place and deploy just by doing a git push;
-
Node.js for the backend, as I’m learning it and I want to keep exercising. Not to mention that is very common and very scalable;
-
Azure SQL as I want to have a database ready for anything I may want to throw at it;
I searched in the TodoMVC and TodoBackend but didn’t find this specific stack of technologies…so why not creating it myself, I thought? Said and done! Here’s some notes I took while building this.
Azure Static Web Apps
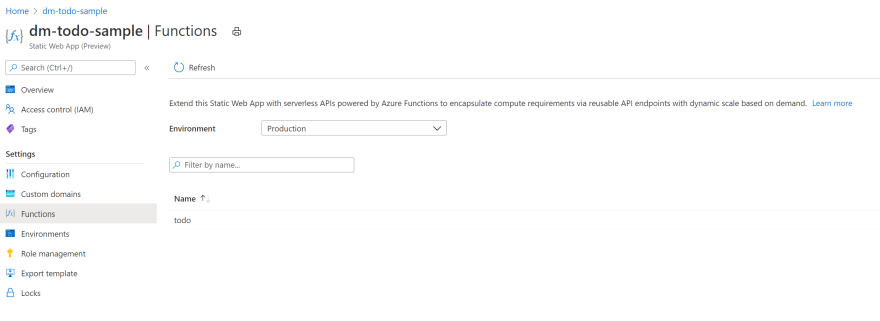
Still in Preview but I loved it as soon as I saw it. Is just perfect for a full-stack development experience. In one shot you can deploy front-end and back-end, make sure they are correctly configured to work together (you know, CORS) and correctly secured.
Deployment is as easy as configuring a GitHub Action, that is actually automatically done for you, even if you still have full access to it, so you can customize it if needed (for example to include the database in the CI/CD process).
Azure Static Web Apps will serve a static HTML whatever you specify as the app
and will spin up and deploy an Azure Function using Node.js to run the back-end using anything you instead specify as the api
:

As you can guess from the configuration, my repo contains the front-end in the client
folder and the back-end code in the api
folder:
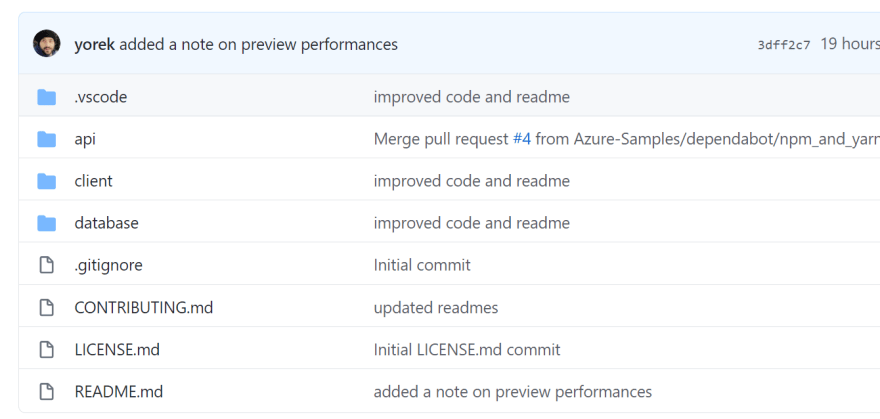
Front-End: Vue.js
As I’m still learning also Vue I kept the code very simple and actually started from the TodoMVC Vue sample you can find on the Vue website: TodoMVC Example.
I like this sample a lot as it shows the power of Vue.js using a single file. Very easy to understand if you have just started learning it. If you are already an experienced Vue user, you’ll be happy to know the Azure Static Web Apps has a native support for Vue, so that you can build and deploy Vue CLI. I’m honestly not that expert yet so I really like the super-simple approach that Vue also offers. Plus I also think that the super-simple approach is perfect for learning, which make it just great for this post.
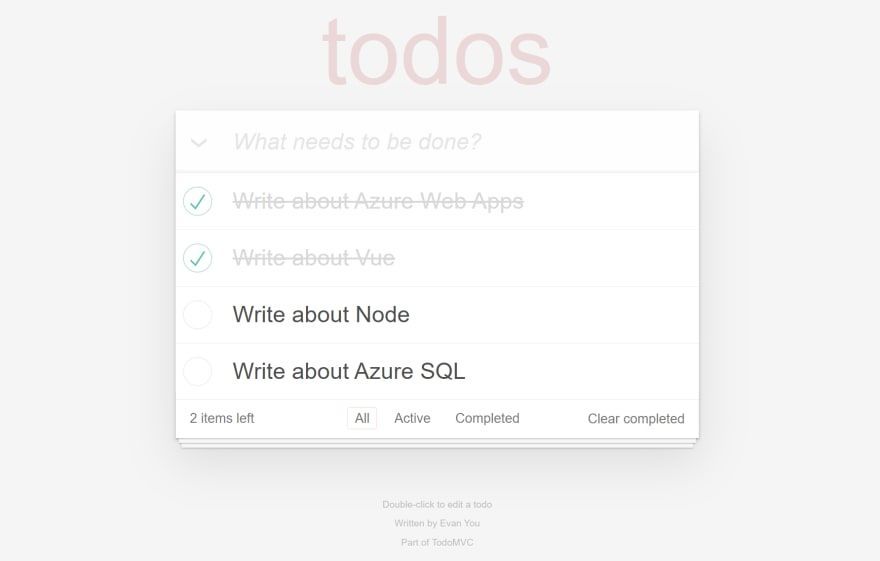
Call a REST API
The original TodoMVC sample uses a local storage to persist To-Do data. Thanks to the Watchers feature that Vue provides, the code JavaScript code you need to write is very simple as any changes to a watched list – todo
in this case – is automatically persisted locally via the following snipped of code:
watch: {
todos: {
handler: function(todos) {
todoStorage.save(todos);
},
deep: true
}
},
Of course, to create a real-world full-stack sample, I wanted to send the To-Do list data to a REST API, avoiding the usage of local storage, to enable more interesting scenarios, like collaboration, synchronization on multiple devices and so on.
Instead of relying on a Watcher, which would unfortunately send the entire list to the REST API and not only the changed item, I decided to go for a more manual way and just call the REST API just binding them directly to the declared methods:
methods: {
addTodo: function () {
var value = this.newTodo && this.newTodo.trim();
if (!value) {
return;
}
fetch(API + "/", {headers: HEADERS, method: "POST", body: JSON.stringify({title: value})})
.then(res => {
if (res.ok) {
this.newTodo = ''
return res.json();
}
}).then(res => {
this.todos.push(res[0]);
})
},
Connecting the addTodo
method to an HTML object is really simple:
<header class="header">
<h1>todos</h1>
<input class="new-todo" autofocus autocomplete="off" placeholder="What needs to be done?" v-model="newTodo"
@keyup.enter="addTodo" />
</header>
With these changes done, it’s now time to take a look at the back-end.
Back-End: Node
Azure Static Web Apps only support Node.js as a backend language today. No big deal, Node.js is a great, fast and scalable language that works perfectly with Azure Function and Azure SQL so we’re really good here. If you are not familiar on how to run Azure Function with Node.js and Azure SQL make sure to read this article: Serverless REST API with Azure Functions, Node, JSON and Azure SQL. As Azure Static Web Apps uses Azure Functions behind the scenes, everything you learned for Azure Function will be applicable to Azure Static Web Apps back-ends.
The client will send a HTTP request to the back-end REST API passing the To-Do payload as JSON. For example to mark a To-Do as done, this JSON
{"completed":true}
will be send via a PUT request:
https://xyz.azurestaticapps.net/api/todo/29
to set the To-Do with Id 29 as done. If everything is ok the REST API will return the entire object, to make sure the client always have the freshest data:
[{
"id":29,
"title":"Write about Vue",
"completed":1
}]
Thanks to Azure SQL support to JSON, the back-end doesn’t have to do a lot…just turn an HTTP request into a call via the TDS protocol supported by Azure SQL but beside that there isn’t a lot to do. JSON will be passed as is, so what the back-end really has to do is to make sure that depending on the HTTP request method invoked, the correct Azure SQL operation will be executed. For example a PUT request should call and UPDATE statement. Implementation is very easy:
switch(method) {
case "get":
payload = req.params.id ? { "id": req.params.id } : null;
break;
case "post":
payload = req.body;
break;
case "put":
payload = {
"id": req.params.id,
"todo": req.body
};
break;
case "delete":
payload = { "id": req.params.id };
break;
}
If you have more complex needs you may decide to implement one function per HTTP request method, but it this case would have been an overkill. I really try to follow the KISS principle as much as possible. The simple the better. But not simpler! (Of course if that would be production code I would check and make sure that JSON is actually valid and harmless before passing it to Azure SQL. Never trust user-provided input, you never know!)
Database: Azure SQL
Azure SQL has been created with just one simple table:
create table dbo.todos
(
id int not null primary key
default (next value for [global_sequence]),
todo nvarchar(100) not null,
completed tinyint not null
default (0)
)
As a developer I still prefer to use JSON in the backend and to send data back and forth to Azure SQL, so that I can also minimize the roundtrips and thus improve performances, so all the stored procedures I’m using have this very simple signature:
create or alter procedure [web].[get_todo]
@payload nvarchar(max)
Then inside the stored procedure I can then use OPENJSON
or any of the JSON functions to manipulate JSON. This way it becomes really easy to accept “n” To-Do as input payload. For example, let’s say I want to delete three To-Dos at once. I can pass something like
[{"id":1}, {"id":2}, {"id":8}]
and then just by writing this
delete t from dbo.todos t
where exists (
select p.id
from openjson(@payload) with (id int) as p where p.id = t.id
)
I can operate on all the selected To-Dos at once. Super cool, and super fast! The ability of Azure SQL to operate both with relational and non-relational features is really a killer feat!
Why Azure SQL and not a NoSQL database?
Answering that question could take a book so let me try to summarize. A NoSQL database for a To-Do list app is more than enough. But I always try to think about future improvements, and I want to make sure than anything I’d like to do in future will be reasonably well supported by my database. I might need to have geospatial data, to aggregate data to do some analytics, I may want to use graph or I may need to create a concurrent system to allow more than one person working on he same to-do list and I need a structure without locks. All these things are available inside Azure SQL without requiring me to use anything other than a technology I already know. This means that I’ll be super productive. I won’t even have scalability issues as with Azure SQL I can go up to 100 TB.
A To-Do list has a pretty well-defined schema, and the performance I can get out of a properly designed relational database are exceptional and cover a huge spectrum of use cases. With a NoSQL database I might squeeze a bit more performances when I focus on a very specific use case, but at the expense of all the others. I really want to keep door open to any improvement so, for this time, for my use case and future needs, I think Azure SQL is the best option I have here.
Keep in mind that well-defined schema doesn’t mean carved in stone. I can have all the flexibility I may want as I can easily store To-Do as JSON (or just a part of it) into Azure SQL, mixing relational and non-relational features, allowing end-users to add custom field and properties if the want to. Actually, you know what? That looks like a great idea for a post. I’ll definitely write on on this topic, so stay tuned!
Conclusion
Creating and deploying a full-stack solution is really easy now, thanks to Azure Static Web Apps. Completely serverless, you can just focus on coding and design while enjoying the simplicity – along with scalability and flexibility – that serverless solution offers. Azure SQL will guarantee that your solution is future-prof, providing scalability out and up to 100 TB with all the perks of a modern post-relational database, like multi-model support, security built-in, columnstore, lock-free tables and anything you may need in your wildest dream.
As usual enjoy the full source code here: https://github.com/Azure-Samples/azure-sql-db-todo-mvc
by Contributed | Sep 24, 2020 | Azure, Technology, Uncategorized
This article is contributed. See the original author and article here.
To help users be even more productive with Azure Synapse Analytics, we are introducing the Knowledge center in the Azure Synapse Studio. You can now create or use existing Spark and SQL pools, connect to and query Azure Open Datasets, load sample scripts and notebooks, access pipeline templates, and tour the Azure Synapse Studio − all from one place!
The Knowledge center can be accessed through the Azure Synapse Studio via both “Useful links” in the bottom right of the Homepage on the main navigation and via the “?” icon in the header.

Use samples immediately
The Knowledge center offers several one-click tutorials that create everything you need to instantaneously explore and analyze data.
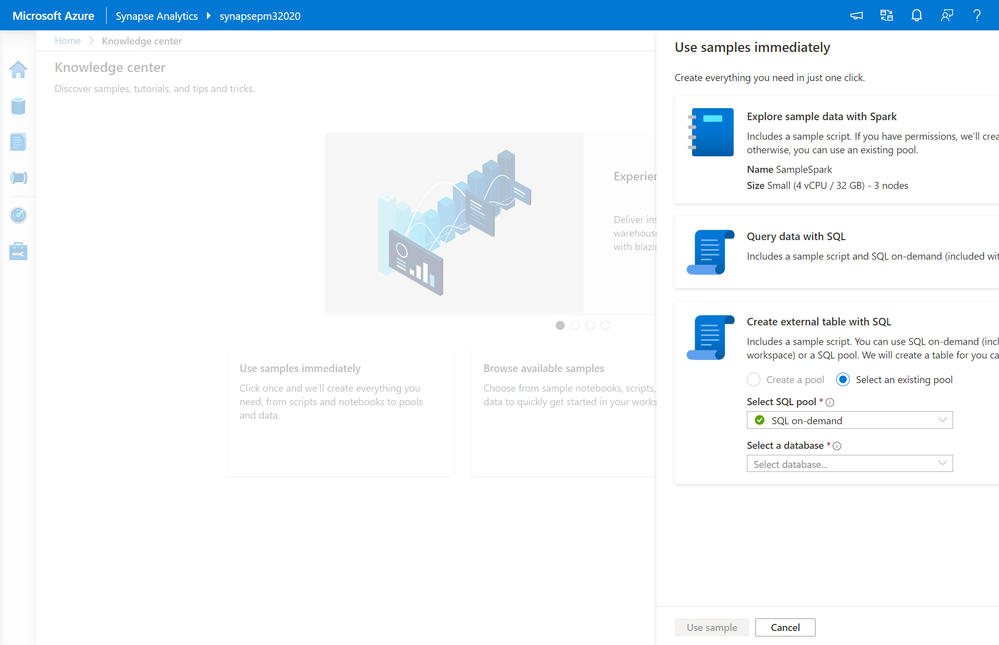
In the “Explore sample data with Spark” tutorial, you can easily use Apache Spark for Azure Synapse to ingest New York City (NYC) Yellow Taxi data and then use notebooks to analyze the data and customize visualizations.
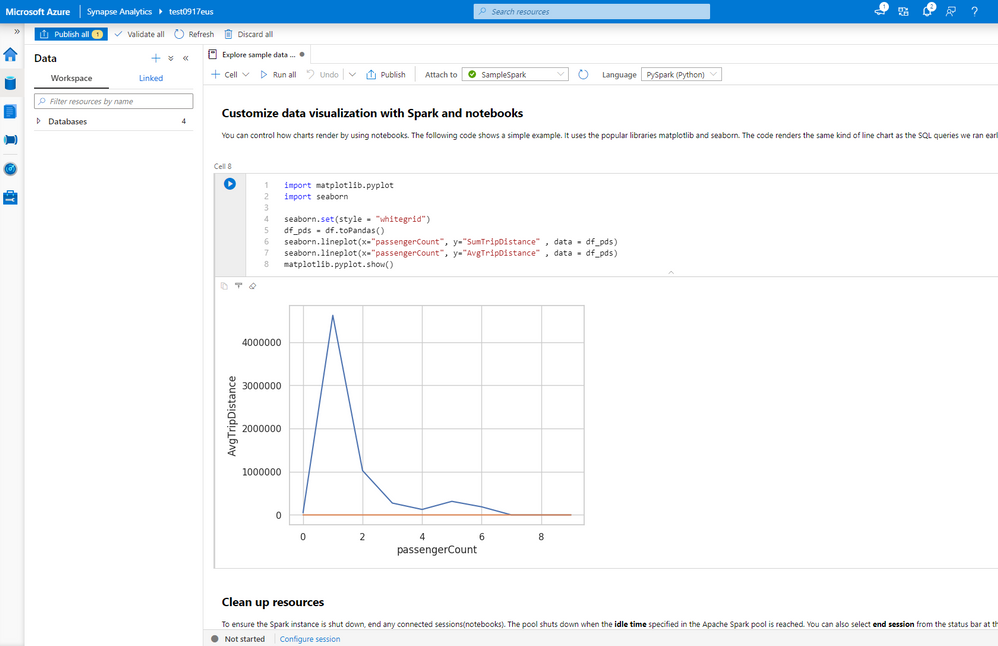
In the “Query data with SQL” tutorial, you can query and analyze data from the NYC Yellow Taxi dataset with a serverless SQL pool, which allows you to use T-SQL for quick data lake exploration without provisioning any additional resources. The tutorial also enables you to quickly visualize results with one click.
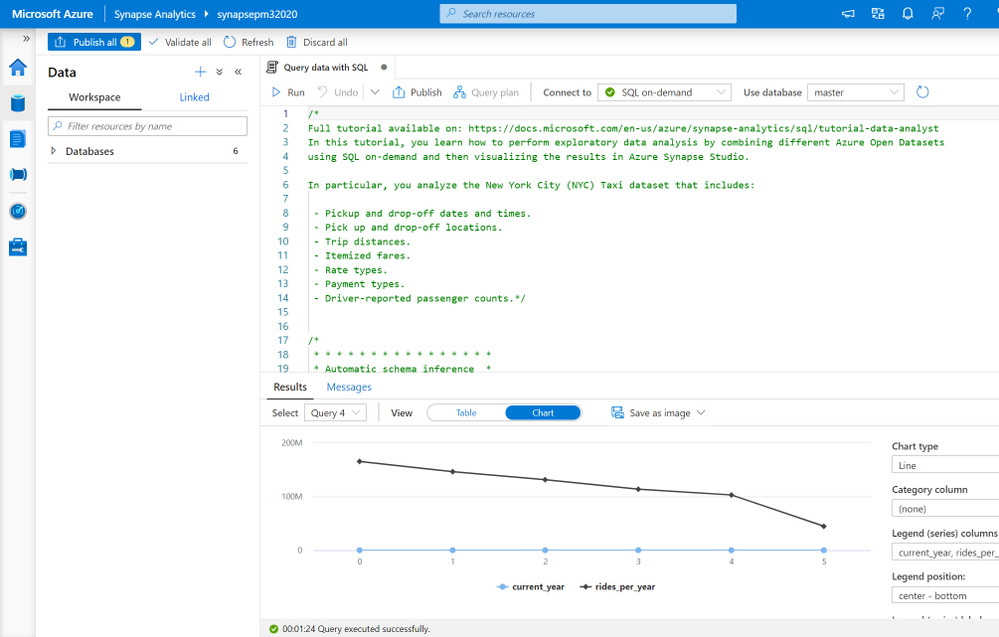
The “Create external table with SQL” tutorial allows you to use either a serverless or dedicated SQL pool to create an external table.
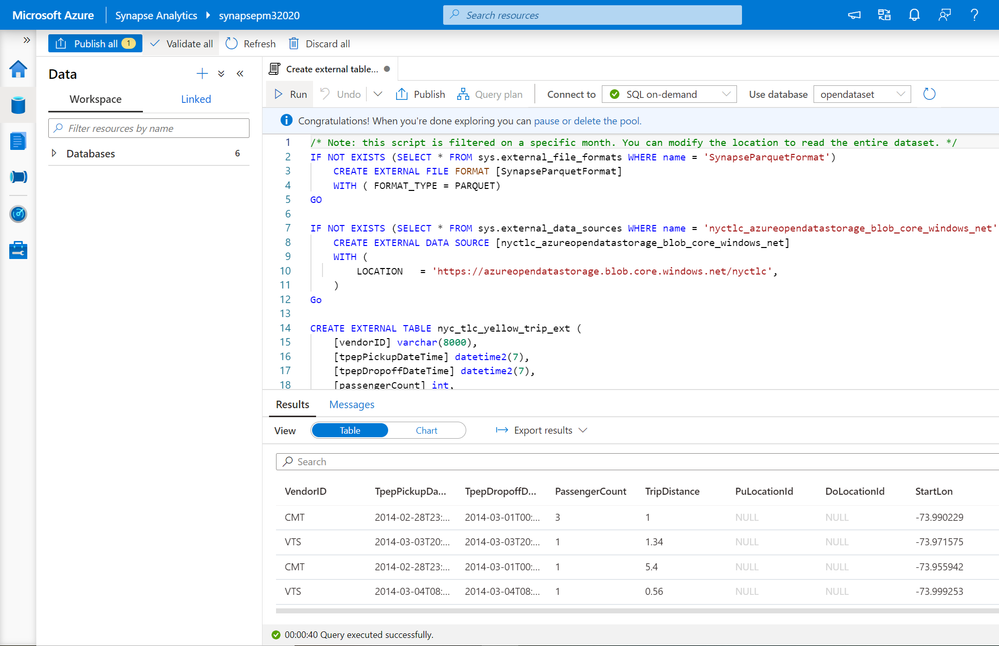
Browse available samples
The new Knowledge center also contains numerous sample datasets, notebooks, scripts, and pipeline templates to allow you to quickly get started. Add and query sample data on COVID-19, public safety, transportation, economic indicators, and more. Regardless of whether you prefer to use PySpark, Scala, or Spark.NET C#, you can get started using a variety of sample notebooks. In addition to sample notebooks, there are samples for SQL scripts like “Analyze Azure Open Datasets using SQL On-demand,” “Generate your COPY Statement with Dynamic SQL,” and “Query CSV, JSON, or Parquet files” along with more than 30 templates for pipelines.
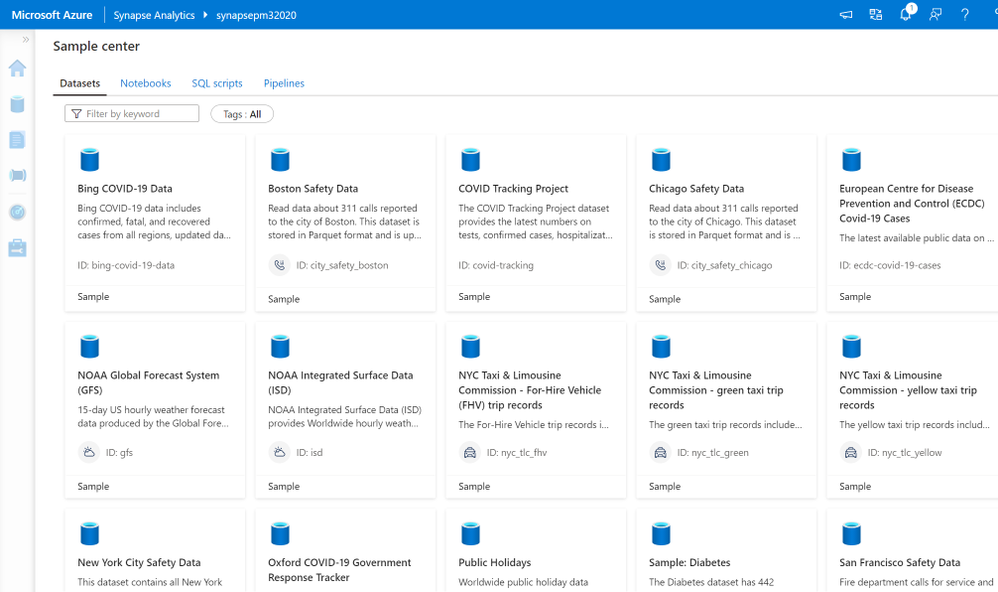
Tour Azure Synapse Studio
The Knowledge center offers a comprehensive tour of the Azure Synapse Studio to help familiarize you with key features so you can get started right away on your first project!
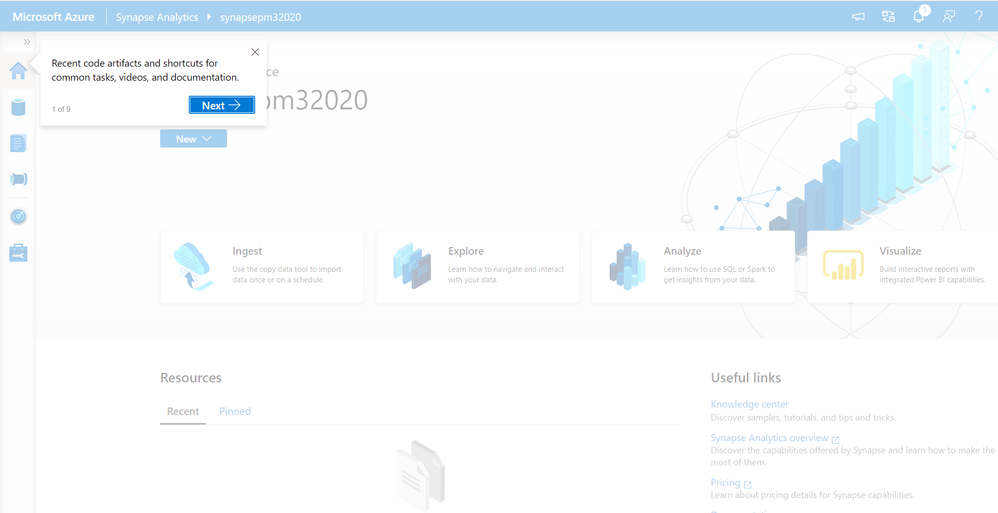
Try the Knowledge center today
by Contributed | Sep 24, 2020 | Uncategorized
This article is contributed. See the original author and article here.
In the past 6 months, I’ve spoken to customers around the world about the challenges associated with providing secure and seamless access for a remote workforce. Organizations need to maximize user productivity while safeguarding the business from cyber threats, but they also must reduce costs in light of today’s difficult economic conditions. To help you meet these goals, Microsoft announced several new product enhancements for Ignite 2020. But we can’t go at it alone. Partnerships play a key role in complementing our built-in capabilities. Today, I’d like to share 7 key ways solutions from partners working with Microsoft enable a secure, productive workforce.
Simplifying identity management and access to your apps
Software-as-a-service (SaaS) and cloud-based apps have been key enablers of user productivity—especially with so many people working from home. Out of the box, Azure AD integrates with leading SaaS apps, with more added every month. These integrations simplify user lifecycle management and app provisioning, allowing you to automatically create and update user identities and roles. Adobe and ServiceNow are two partners that we’ve developed integrations that can ensure employees have access to the right applications through their tenure at your organization.
Adobe announces support for SCIM-based provisioning.
To streamline access and administration of its business-critical apps, Adobe has announced a SCIM standard-based app provisioning integration for its core Adobe Identity Management platform. Working with Microsoft IT as a customer to get insights, Adobe has built an updated admin experience, which will make it easier to manage user lifecycles across Adobe Creative Cloud, Adobe Document Cloud, and Adobe Experience Cloud. This integration will be available for limited preview in October and generally available for customers by the end of 2020.
Screenshot of updated Adobe Admin experience to enable SCIM provisioning with Azure AD. Experience subject to change.
ServiceNow integrates with Azure AD to automate new hire onboarding
ServiceNow recently announced in their latest Now Platform Paris release new capabilities to automatically kick off the right onboarding workflows as soon as a new employee profile is created in Azure AD. IT and hiring managers can automatically provision application access for new hires through Azure AD, including from an HR system, increasing productivity for employees and support teams. This integration automates the whole onboarding workflow from case creation in ServiceNow HR Service Delivery, to role assignment by hiring manager, and application provisioning by IT based on the new hire’s role. Learn more about ServiceNow and Azure AD’s new employee onboarding capabilities.
Saviynt is partnering with Azure AD to provide advanced identity governance capabilities to customers
Saviynt is working with Microsoft and Azure AD to provide additional governance scenarios to customers. Saviynt Cloud Privileged Access Management (PAM) now integrates with Azure AD Privileged Identity Management and Identity Protection to create an identity led, Zero Trust security service to accelerate an enterprise’s digital transformation journey. Saviynt Cloud PAM has also extended their solution to provide privileged access for Microsoft Azure IaaS and expanded governance to Azure AD B2C customers (public preview coming soon). In the recent update to the Saviynt for Microsoft Teams governance, the solution now provides Microsoft Teams site succession management and support for Teams Private Channels. Learn more about the Azure AD and Saviynt partnership.
Enabling stronger security through passwordless, identity verification, and threat intelligence
With more employees working from home, we know that security is even more top of mind. This starts with securing identities. Azure AD capabilities like passwordless are designed to help protect identities with minimal impact to employees. Security operations (SecOps) teams also need greater visibility to enable them to take the right actions in remediating threats. Several recent partnerships have helped us advance these goals.
Illusive Networks integrates with Microsoft Security and Azure AD APIs
Illusive Networks enhances the visibility and monitoring of vulnerable privileged identities in Azure AD, such as redundant identities, identities with excessive privileges, risky practices (e.g. Azure MFA disabled), and unauthenticated identities. Learn more about Illusive Networks’ new integrations across Microsoft Security products.
Yubico enables the move to passwordless
Weak passwords are the most vulnerable attack vector, which is why we are such strong advocates of passwordless technologies. To help reduce the reliance on passwords, we’ve developed a limited time offer with Yubico where qualified services partners can nominate their customers to go passwordless. Learn more about the new program and ways we’re partnering with Yubico in the video below.
Enabling Identity Proofing and Verification capabilities to Azure AD B2C through partners
As more businesses move to online, they need to verify and onboard customers remotely. Jumio and Onfido now enable Azure AD B2C customers to perform identity card (passport or driver license) scanning, identity verification, and liveness detection during a user’s journey.
Protect legacy applications through new secure hybrid access partnerships
During the COVID-19 outbreak, our customers need to access all mission critical apps from home securely, including legacy applications. While Azure AD Application Proxy can provide remote access to your legacy apps, we know that some customers prefer to use their existing application delivery networks, VPNs, or Software Defined Perimeter solutions. That’s why we’re expanding our Secure Hybrid Access Partnerships to include new partners such as Kemp, Palo Alto Networks, Cisco AnyConnect, Fortinet and Strata and Ping Identity for Azure AD B2C customers.
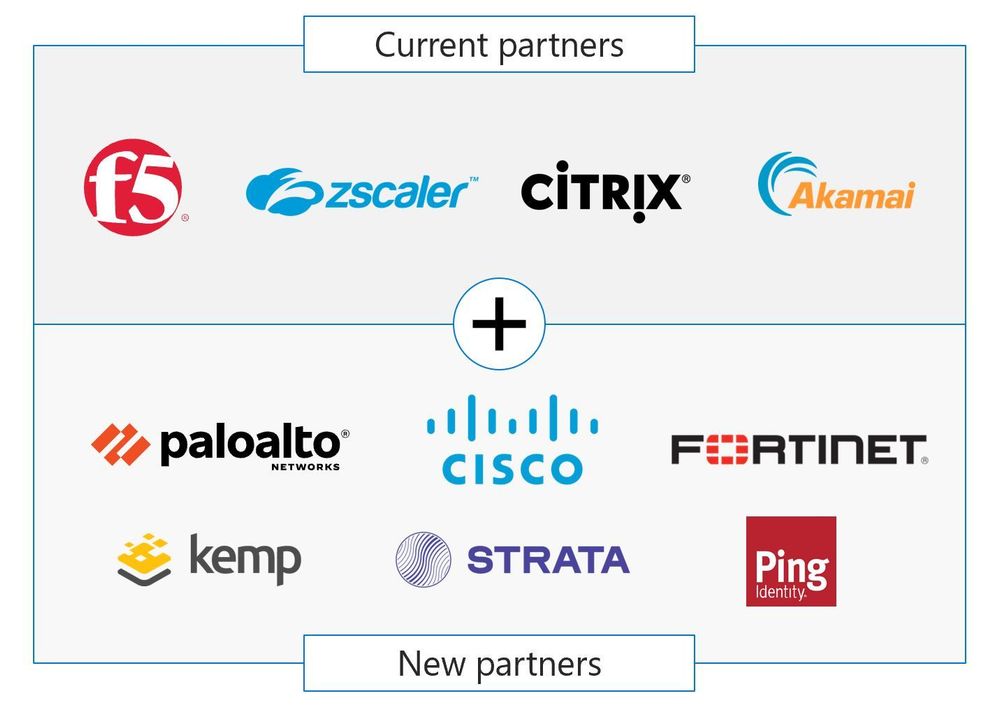
We hope all these announcements are welcome additions as you support the new realities of remote work. Please let us know any feedback you have, including any other partners you think we should be working with to improve the employee experience and security.
Join us virtually, on-demand for Identity Partner Sessions at Ignite 2020
While we wish we could have met in person this year at Microsoft Ignite 2020, we have a great line up of free, virtual sessions to share with you wherever you are in the world. Register for free here.
All the Microsoft Identity sessions, on-demand, can be found on this Microsoft Ignite playlist or the Video Hub. Here are my top sessions to attend that relate to our partner solutions:
- Azure Active Directory: our vision and roadmap to help you secure remote access and boost employee productivity
- Save money by securing access to all your apps with Azure Active Directory
- Bridge the gap between HR, IT and business with Azure Active Directory
- Build experiences that customers and partners will love with Azure Active Directory External Identities
Best regards,
Sue Bohn
Partner Director of Program Management
Microsoft Identity Division
by Contributed | Sep 24, 2020 | Azure, Technology, Uncategorized
This article is contributed. See the original author and article here.
Hi Everyone,
This week we have announced the availability of the initial public preview of Azure Kubernetes Service (AKS) on Azure Stack HCI.
You can evaluate AKS on Azure Stack HCI by registering for the Public Preview here: https://aka.ms/AKS-HCI-Evaluate
Azure Kubernetes Service on Azure Stack HCI takes our popular Azure Kubernetes Service (AKS) and makes it available to customers to run on-premises; delivering Azure consistency, a familiar Azure experience, ease of use and high security for their containerized applications. AKS on Azure Stack HCI enables developers and administrators to deploy and manage containerized apps on Azure Stack HCI. You can use AKS on Azure Stack HCI to develop applications on AKS and deploy them unchanged on-premises, run Arc enabled Data Services on a resilient platform and modernize Windows Server and Linux applications.
With AKS on Azure Stack HCI, Microsoft is delivering an Industry leading experience for modern application development and deployment in a hybrid cloud era. Microsoft is the only company that delivers technology that takes you from bare metal to a public cloud connected and consistent application and data platform in your datacenter.
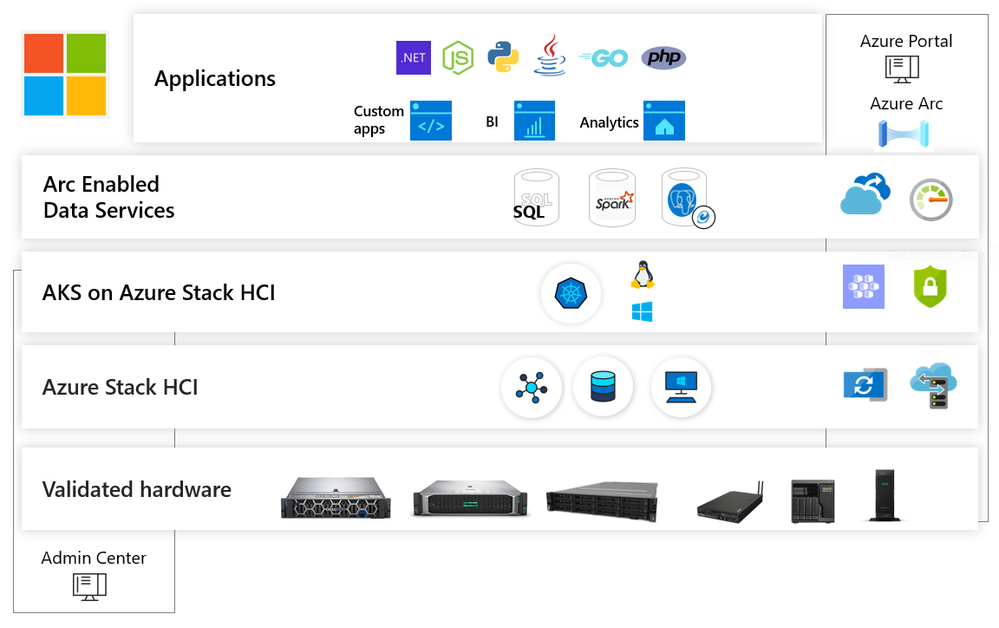
AKS on Azure Stack HCI can run Windows and Linux containers, all managed and supported by Microsoft. AKS on Azure Stack HCI leverages our experience with AKS, follows the AKS design patterns and best-practices, and uses code directly from AKS. This means that you can use AKS on Azure Stack HCI to develop applications on AKS and deploy them unchanged on-premises. It also means that any skills that you learn with AKS on Azure Stack HCI are transferable to AKS as well.
AKS on Azure Stack HCI uses Windows Admin Center and PowerShell to provide an easy to use and familiar deployment experience for any user of Azure Stack HCI. AKS on Azure Stack HCI simplifies the process of setting up Kubernetes on Azure Stack HCI and includes the necessary components to allow you to deploy multiple Kubernetes clusters in your environment.
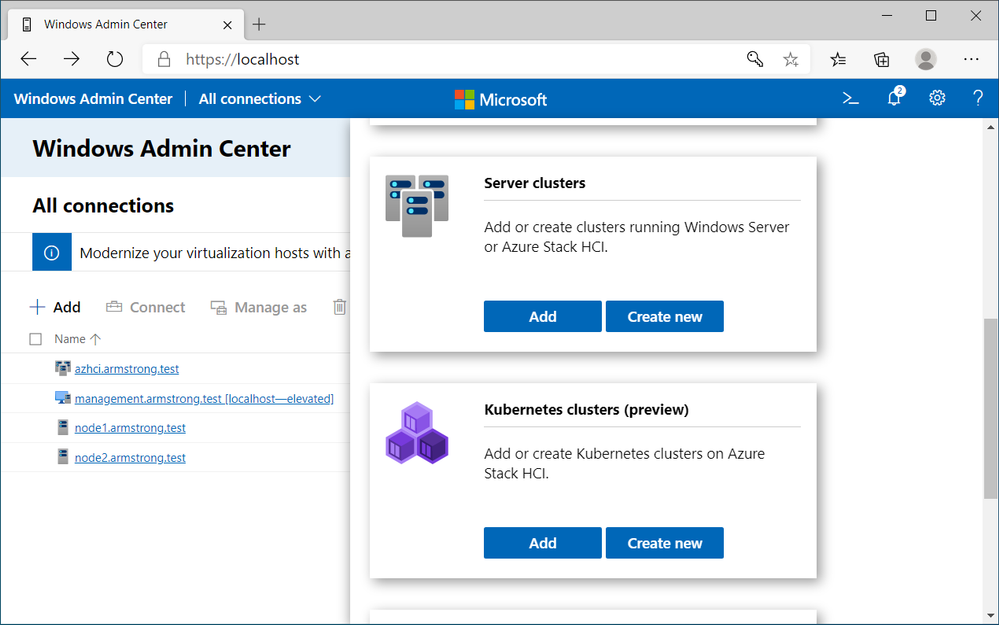
Which all means that you can focus on what matters most to you – your applications.
AKS on Azure Stack HCI is designed such that every layer is secure. Microsoft provides a secure baseline of all components in AKS on Azure Stack HCI and keeps them up to date. We will be adding mode security features and further hardening the platform over the course of the public preview.
AKS on Azure Stack HCI fully supports both Linux-based and Windows-based containers. When you create a Kubernetes cluster on Azure Stack HCI you can choose whether to create node pools (groups of identical virtual machine, like on AKS) to run Linux containers, Windows containers, or both. AKS on Azure Stack HCI creates and maintains these virtual machines so that you don’t have to directly manage operating systems.
If you have existing .NET applications that you want to modernize, and take advantage of the latest cloud development patterns, AKS on Azure Stack HCI is the platform for you. AKS on Azure Stack HCI provides an industry leading experience for Windows Containers on Kubernetes. We are also working on great tooling and documentation for the process of moving .NET applications from virtual machines to containers with AKS on Azure Stack HCI.
If you are building a new cloud native applications on AKS, AKS on Azure Stack HCI provides to easiest way for you to take those applications and run them in your datacenter. AKS on Azure Stack HCI shares a common code base with AKS, the user experience is consistent across both products, and Microsoft is investing to ensure that applications can move easily between these two environments.
If you are wanting to utilize new Microsoft technologies like Arc enabled Data Services in your datacenter, AKS on Azure Stack HCI delivers a complete solution from Microsoft. It is validated and supported by Microsoft, designed to deliver the best experience for these applications.
You can learn more about AKS on Azure Stack HCI by watching:
Working on this project has been a lot of fun for everyone involved, and we are excited to finally be able to share this with the world. I look forward to seeing what everyone is able to achieve with AKS on Azure Stack HCI!
Cheers,
Ben Armstrong
Recent Comments